In order to allow Utopian contributors to design LHC analysis codes that could be helpful to particle physics, it is first necessary to explain what one detects in a collider experiment such as those ongoing at the Large Hadron Collider (the LHC) at CERN. In addition, it is also needed to be able to link those detectable physics objects to the manner to implement and use them in the MadAnalysis 5 platform that we plan to use (that is also mirrored on GitHub).
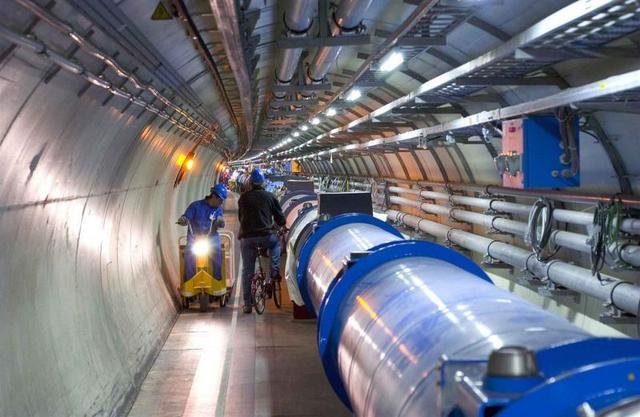
[image credits: CERN]
This post thus consists in the step 1a of the roadmap sketched here.
I decided to split the initial step 1 into several parts, as I prefer to move slowly and strongly instead of quickly and unreliably.
Each post will hence go straight to one specific point instead of plenty of them. I want to somehow make sure that everyone follows.
They are several classes of objects that are reconstructed in a typical LHC detector. They are classified through six containers in the MadAnalysis 5 framework (we will focus on only three of them today).
The handling of those objects is crucial for the rest of this project, and I will therefore propose a series of programming exercises accompanying my posts to facilitate their usage (in a way in which one understands what one is doing). I believe those exercises are important for a better handling of the next more complicated (at least from the physics standpoint) steps.
I invite all the participants to publish the pieces of code solving the exercises on Utopian. Please make sure to tag me either directly on the post or on discord. My discord username is lemouth#8260, and I can be found both on the steemstem and utopian discord servers. Without such a mention, I may just miss your post :/
I am obviously available for questions (both on discord and in the comment section of this article).
PARTICLE DETECTION AT THE LHC IN A NUTSHELL
In a particle collision such as those on-going at the LHC, many particles are produced.
Some of them can reach the material of the detectors unaltered, so that they can interact with it. This leaves signals that allow physicists to infer the presence of these particles (through an often complex electronic apparatus). This is what we call stable particles, as they are stable on detector time scales.
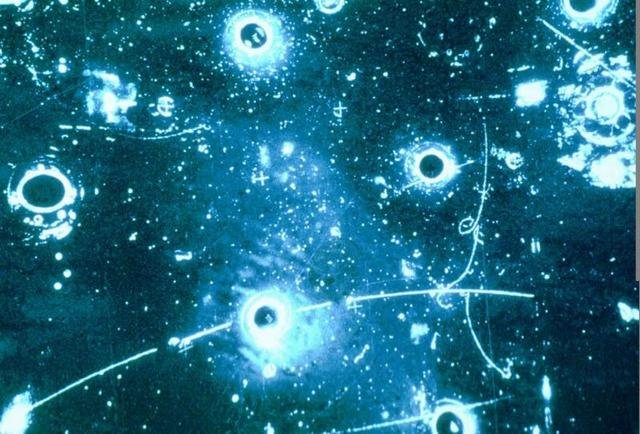
[image credits: CERN]
The second class of objects that can be reconstructed in a detector are what we call jets of strongly-interacting particles, or simply jets.
The strong interaction is one of the three fundamental interactions and it plays a key role at the LHC. It indeed governs the LHC collision processes from almost A to Z.
Of course, we are here more interested in the Z side, that tells us that any produced strongly-interacting particle always leads to a jet made of many of them.
I will skip any extra detail for today; I hope to have been clear enough in a small amount of words. But please do not worry, I will come back to this very soon.
The last class of objects that can be reconstructed in a detector consists of unstable particles. As their name suggest it, those particles decay, almost instantaneously and through a sometimes complicated process, into stable particles and/or jets.
Therefore, one cannot detect them, and only the analysis of the properties of the observed final-state particles allows to infer their initial presence.
And of course, we are also capable to detect the invisible… This may be seen as a fourth class of observable objects (yes, really!) in the detector records of any LHC collision: the missing energy.
THE STRUCTURE OF A MADANALYSIS 5 ANALYSIS
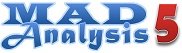
[image credits: MadAnalsysis 5]
It is now time to make a parallel with the MadAnalysis 5 framework.
Instead of providing a full manual that one may have forgotten before starting anything more concrete, I will instead be practical and proceed with a series of well defined exercises, closely linking physics to coding.
One given collision recorded in a detector is called an event. Correspondingly, the corresponding object in the MadAnalysis 5 framework is called event
.
The LHC analyses that will be implemented within this Utopian project hence consist in C++ codes allowing to process the information included in an entire sample of event
objects, representing what could really happen in thousands of LHC collisions.
In practice, the task that will be assigned to the contributors will be to compute quantities that will allow one to make decisions about how to treat an event. One indeed needs to decide if one needs to reject an event (because it is found boring) or to keep it for further investigations (as a source of a potentially interesting phenomenon).
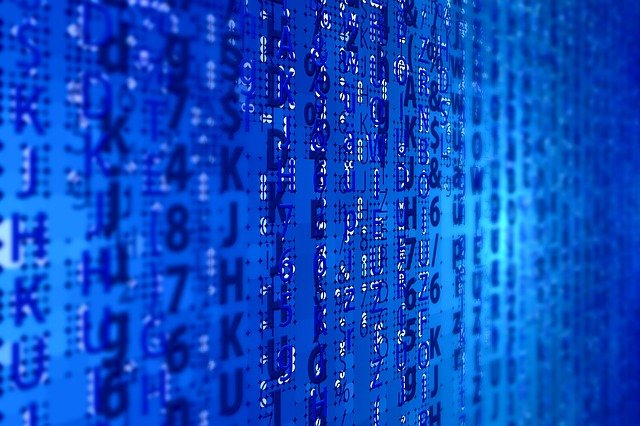
[image credits: Pixabay (CC0)]
In order to start, one should go to the directory in which MadAnalysis 5 has been installed (see here for the installation instructions) and then type, in a shell,
./bin/ma5 -E test_folder test_analysis
This leads to the creation of a folder named test_folder
in which we will code a toy analysis (i.e. the exercise to be done in this post).
The file to modify to this end is
test_folder/Build/SampleAnalyzer/User/Analyzer/test_analysis.cpp
The code inside it contains three methods named Initialize
, Execute
and Finalize
. In this post, we are only interested in modifying the Execute
method.
If one looks into that test_analysis.cpp
file and in the template for the Execute
method, one can observe that it takes an event
object in argument,
bool test_analysis::Execute(SampleFormat& sample, const EventFormat& event)
{
…
}
One will hence have to implement a code processing the attributes of this event
object.
STABLE PARTICLES
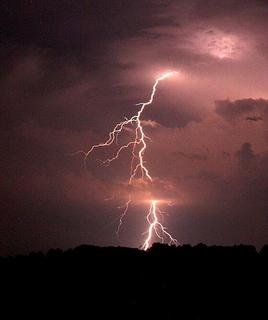
[image credits: Wikimedia]
The first category of particles that I want to focus on consists in the set of particles that are stable from the point of view of a typical LHC detector.
This means that once produced, these particles will just fly until they meet the material of the detector itself.
At that moment, they will interact with this material and leave tracks and/or energy deposits from which physicists will be able to deduce their presence and measure their properties.
There are three of these particles: electrons, muons and photons (as well as the associated antiparticles). In other words, these objects are observed as such and can be reconstructed (with a given efficiency) almost straightforwardly from all their hits in the detector.
All the physics objects that are reconstructed in an event can be accessed through the event
object attached to each collision event:
- electrons are stored in the
event.rec()->electrons()
container; - muons are stored in the
event.rec()->muons()
container; - photons are stored in the
event.rec()->photons()
container.
Kind of straightforward isn’t it? The three above C++ objects are vectors of special objects with given properties. The study of these properties will be the topic of the next post (this one is already way too long), as well as the investigation of any other type of objects recorded in a detector.
But in short, one can implement a loop over these vectors like for any c++ vector,
for(unsigned int ii=0; ii<event.rec()->electrons().size(); ii++)
{
…
}
THE EXERCISE
Let us first play a little bit with electrons, photons and muons. As I do no know the level of the participants to this project, I propose a super easy exercise. For more complicated things, please stay tuned! :)
Download a sample of 10 simulated LHC collisions from here. This file has been generated by means of the Delphes program that has been installed together with MadAnalysis 5, under the root format (also installed last week). I repeat that information on the installation procedure can be found here.
Edit the file
test_folder/Build/SampleAnalyzer/User/Analyzer/test_analysis.cpp
and implement a piece of code in theExecute
function allowing for the evaluation of the numbers of photons, electrons and muons present in each event. Following Utopian guidelines, I am expecting all codes to be stored on github.Compile the code and generate an executable by typing
cd test_folder/Build source setup.sh make
Create the (text) file
test_folder/Input/tth_aa.list
and indicate in it the absolute path to the downloaded event file (the location of thetth_aa.root
file on the local machine).Execute the code
cd test_folder/Build; ./MadAnalysis5job ../Input/tth_aa.list
Please provide the answer to the question raised in point 2.
Don’t hesitate to write a post presenting and detailing your code allowing to get to the answer. Please do not forget to mention me directly within your post, so that I could easily find it.
I also kindly ask to commit the c++ source file to this GitHub repository repository so that it would be easier for me to double check what has been done. Please follow the following syntax for the filename:
ex1a-<steemitID>.cpp
I fix the deadline to Wednesday May 30th, 16:00 UTC (I repeat, this exercise is easy). Then we will move on with the next step.
After this easy warm-up, things will get harder and more interesting next week! Remember, the goal is to implement a particle physics analysis such as those really performed at the LHC at CERN!
STEEMSTEM
SteemSTEM is a community-driven project that now runs on Steem for more than 1.5 year. We seek to build a community of science lovers and to make the Steem blockchain a better place for Science Technology Engineering and Mathematics (STEM).
More information can be found on the @steemstem blog, on our discord server and in our last project report. Please also have a look on this post for what concerns the building of our community.